Categorize WordPress Users with Custom Taxonomies Without Plugin


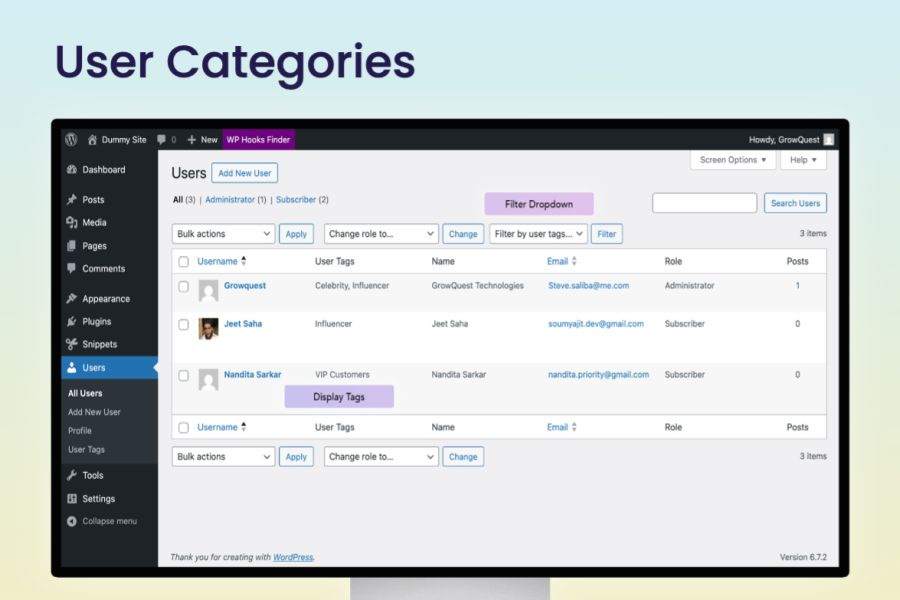
We encountered a unique challenge in one of our recent projects when a client required extensive classification for their registered WordPress users. The client needed to categorize their user base—which included influencers, celebrities, high-tier buyers, and nearly a hundred different user classifications. While WordPress provides built-in user roles for basic categorization, managing too many roles quickly becomes cumbersome and clutters the user management interface. Additionally, an excess number of roles can significantly slow down website performance, especially when using plugins that directly interact with roles for functionalities such as role-based content access or customized menu navigation.
To address this challenge efficiently, we implemented custom taxonomies for WordPress users, streamlining user categorization without overwhelming the management interface or impacting website performance.
Don’t like to read? Here’s a quick link to the complete code solution.
Step 1: Registering a Custom User Taxonomy
The first step was to register a custom taxonomy named “User Tags” for WordPress users, enabling efficient and scalable classification beyond the default user roles.
/**
* Register custom taxonomy 'User Tags' for WordPress users.
*/
function gq_register_user_tag_taxonomy() {
register_taxonomy( 'user_tag', 'user', [
'labels' => [
'name' => _x( 'User Tags', 'Taxonomy General Name', 'growquest' ),
'singular_name' => _x( 'User Tag', 'Taxonomy Singular Name', 'growquest' ),
'menu_name' => __( 'User Tags', 'growquest' ),
'all_items' => __( 'All User Tags', 'growquest' ),
'parent_item' => __( 'Parent User Tag', 'growquest' ),
'parent_item_colon' => __( 'Parent User Tag:', 'growquest' ),
'new_item_name' => __( 'New User Tag', 'growquest' ),
'add_new_item' => __( 'Add New User Tag', 'growquest' ),
'edit_item' => __( 'Edit User Tag', 'growquest' ),
'update_item' => __( 'Update User Tag', 'growquest' ),
'view_item' => __( 'View User Tag', 'growquest' ),
'add_or_remove_items' => __( 'Add or Remove User Tags', 'growquest' ),
'choose_from_most_used' => __( 'Choose from Most Used User Tags', 'growquest' ),
'popular_items' => __( 'Popular User Tags', 'growquest' ),
'search_items' => __( 'Search User Tags', 'growquest' ),
'not_found' => __( 'Not Found', 'growquest' ),
'no_terms' => __( 'No User Tags', 'growquest' ),
'items_list' => __( 'User Tags List', 'growquest' ),
'items_list_navigation' => __( 'User Tags List Navigation', 'growquest' ),
],
'hierarchical' => false, // set to true for categories-like taxonomy, false for tags-like
'public' => false,
'show_ui' => true, // allows management via admin dashboard
'show_admin_column' => false,
'show_in_nav_menus' => false,
'show_tagcloud' => false,
'rewrite' => false,
] );
}
// Hook into WordPress init action.
add_action( 'init', 'gq_register_user_tag_taxonomy' );
Explanation:
This code snippet defines and registers a custom taxonomy
named “User Tags”, allowing site administrators to classify and group registered WordPress users beyond the limitations of default roles. The function gq_register_user_tag_taxonomy()
calls WordPress’s built-in register_taxonomy()
function, associating the taxonomy specifically with the user
object type. It utilizes customized labels and sets hierarchical
to false
, making it behave similarly to tags rather than categories. The taxonomy is configured as non-public, meaning it’s only accessible within the admin area without any public URLs. The function is hooked to the init
action, ensuring the taxonomy is correctly registered during WordPress initialization.
Step 2: Adding the Taxonomy Management Page to the Users Menu
By default, WordPress custom taxonomies do not provide a management UI when assigned to entities other than standard post types
. To address this limitation and manage our user taxonomy effectively, we implemented the following workaround:
/**
* Add 'User Tags' taxonomy management page under the 'Users' menu.
*/
function gq_add_user_tags_admin_page() {
$taxonomy = get_taxonomy( 'user_tag' );
add_users_page(
esc_attr( $taxonomy->labels->menu_name ),
esc_attr( $taxonomy->labels->menu_name ),
$taxonomy->cap->manage_terms,
'edit-tags.php?taxonomy=' . $taxonomy->name
);
}
add_action( 'admin_menu', 'gq_add_user_tags_admin_page' );
/**
* Ensure the 'Users' menu is highlighted when editing 'User Tags' taxonomy terms.
*
* @param string $parent_file Current parent file.
*
* @return string Modified parent file.
*/
function gq_fix_user_tags_parent_file( $parent_file ) {
global $submenu_file;
if ( isset( $_GET['taxonomy'] ) && 'user_tag' === $_GET['taxonomy'] && 'edit-tags.php?taxonomy=user_tag' === $submenu_file ) {
$parent_file = 'users.php';
}
return $parent_file;
}
add_filter( 'parent_file', 'gq_fix_user_tags_parent_file' );
Explanation:
This code snippet achieves two objectives. First, it utilizes the admin_menu
action hook along with add_action()
to create a dedicated admin interface for managing “User Tags” taxonomy terms under the native WordPress Users menu. It does this through the add_menu_page()
functionality.
Second, the code uses the parent_file
filter to ensure the correct admin menu item (“Users”) remains highlighted when editing terms in this custom taxonomy. This enhances the usability and navigation experience in the WordPress admin panel, clearly indicating to administrators their current location within the admin interface.
Step 3: Customizing Admin Columns for User Taxonomy Management
To clearly display user-taxonomy relationships within WordPress’s user management interface, we customize the admin columns by removing irrelevant default columns and adding user-focused taxonomy information. The following code snippet replaces the default “Posts” column with a new “Users” column for the taxonomy, and displays user-assigned taxonomy terms clearly within the admin interface.
/**
* Modify columns for the 'User Tags' taxonomy admin screen.
* Removes the default 'Posts' column and adds a custom 'Users' column.
*
* @param array $columns Existing columns.
*
* @return array Modified columns array.
*/
function gq_manage_user_tag_taxonomy_columns( $columns ) {
// Remove default 'Posts' count column.
unset( $columns['posts'] );
// Add new 'Users' column.
$columns['users'] = __( 'Users', 'growquest' );
return $columns;
}
add_filter( 'manage_edit-user_tag_columns', 'gq_manage_user_tag_taxonomy_columns' );
/**
* Populate custom 'Users' column with user count for each term.
*
* @param string $content Existing column content.
* @param string $column_name The current column name.
* @param int $term_id Term ID.
*
* @return string Modified column content.
*/
function gq_populate_user_tag_taxonomy_column( $content, $column_name, $term_id ) {
if ( 'users' === $column_name ) {
$term = get_term( $term_id, 'user_tag' );
if ( $term && ! is_wp_error( $term ) ) {
$content = esc_html( $term->count );
} else {
$content = '0';
}
}
return $content;
}
add_filter( 'manage_user_tag_custom_column', 'gq_populate_user_tag_taxonomy_column', 10, 3 );
/**
* Adds a 'User Tags' column to the main WordPress Users admin page.
*
* @param array $columns Existing columns in Users admin.
*
* @return array Modified columns with 'User Tags' added.
*/
function gq_add_user_tags_column( $columns ) {
// Insert 'User Tags' after the 'Username' column.
$offset = array_search( 'username', array_keys( $columns ), true ) + 1;
return array_slice( $columns, 0, $offset, true )
+ [ 'user_tags' => __( 'User Tags', 'growquest' ) ]
+ array_slice( $columns, $offset, null, true );
}
add_filter( 'manage_users_columns', 'gq_add_user_tags_column' );
/**
* Display assigned 'User Tags' terms for each user in the custom column.
*
* @param string $output Default column output.
* @param string $column_name Current column name.
* @param int $user_id The user ID.
*
* @return string Modified column content.
*/
function gq_populate_user_tags_column( $output, $column_name, $user_id ) {
if ( 'user_tags' === $column_name ) {
$terms = wp_get_object_terms( $user_id, 'user_tag' );
if ( ! empty( $terms ) && ! is_wp_error( $terms ) ) {
$names = wp_list_pluck( $terms, 'name' );
$output = implode( ', ', $names );
} else {
$output = '—';
}
}
return $output;
}
add_filter( 'manage_users_custom_column', 'gq_populate_user_tags_column', 10, 3 );
Explanation:
The provided code snippet modifies the default WordPress taxonomy admin interface by customizing columns for improved clarity. It first removes the irrelevant default “Posts” column and introduces a new custom column titled “Users,” clearly showing the number of users associated with each taxonomy term. Additionally, it enhances the WordPress Users listing by introducing a new column, “User Tags,” that clearly displays the taxonomy terms assigned to each user. This approach significantly improves the readability and usability of user taxonomies in the WordPress admin dashboard.
Step 4: Displaying and Saving User Tags on the User Profile Page
Because WordPress doesn’t support a management UI for user-assigned taxonomies by default, we need to manually add the functionality to assign taxonomy terms to users from the profile screen and save them accordingly.
/**
* Display 'User Tags' multi-select field on user profile edit screen.
*
* @param WP_User $user The current WP_User object.
*/
function gq_display_user_tags_field( $user ) {
$taxonomy = get_taxonomy( 'user_tag' );
if ( ! current_user_can( $taxonomy->cap->assign_terms ) ) {
return;
}
$user_tags = get_terms( [ 'taxonomy' => 'user_tag', 'hide_empty' => false ] );
?>
<h3><?php esc_html_e( 'User Tags', 'growquest' ); ?></h3>
<table class="form-table">
<tr>
<th><label for="user_tag_select"><?php esc_html_e( 'Select User Tags', 'growquest' ); ?></label></th>
<td>
<?php if ( ! empty( $user_tags ) && ! is_wp_error( $user_tags ) ) : ?>
<select name="user_tag[]" id="user_tag_select" multiple style="min-width:250px;">
<?php
foreach ( $user_tags as $tag ) :
$selected = false;
// If editing an existing user
if ( is_object( $user ) && ! empty( $user->ID ) ) {
$selected = is_object_in_term( $user->ID, 'user_tag', $tag->term_id );
}
?>
<option value="<?php echo esc_attr( $tag->term_id ); ?>" <?php selected( $selected ); ?>>
<?php echo esc_html( $tag->name ); ?>
</option>
<?php endforeach; ?>
</select>
<?php else : ?>
<p><?php esc_html_e( 'No user tags found.', 'growquest' ); ?></p>
<?php endif; ?>
</td>
</tr>
</table>
<?php
}
add_action( 'user_new_form', 'gq_display_user_tags_field' );
add_action( 'show_user_profile', 'gq_display_user_tags_field' );
add_action( 'edit_user_profile', 'gq_display_user_tags_field' );
/**
* Save the selected 'User Tags' when user profile is updated.
*
* @param int $user_id The ID of the current user being edited.
*/
function gq_save_user_tags_field( $user_id ) {
$taxonomy = get_taxonomy( 'user_tag' );
// Permission checks
if ( ! current_user_can( 'edit_user', $user_id ) || ! current_user_can( $taxonomy->cap->assign_terms ) ) {
return;
}
// Assign selected terms
if ( ! empty( $_POST['user_tag'] ) ) {
$terms = array_map( 'absint', $_POST['user_tag'] );
wp_set_object_terms( $user_id, $terms, 'user_tag', false );
} else {
// Remove existing terms if none selected
wp_delete_object_term_relationships( $user_id, 'user_tag' );
}
}
add_action( 'user_register', 'gq_save_user_tags_field' );
add_action( 'personal_options_update', 'gq_save_user_tags_field' );
add_action( 'edit_user_profile_update', 'gq_save_user_tags_field' );
Explanation:
The above code adds a custom multi-select field to WordPress user profile screens—both for editing existing users and creating new ones. It retrieves available taxonomy terms using get_terms()
and checks existing term assignments with is_object_in_term()
. Upon saving the user profile, the function securely updates taxonomy assignments with wp_set_object_terms()
or removes them with wp_delete_object_term_relationships()
. Proper permissions are validated using current_user_can()
to ensure security and integrity.
Step 5: Adding a User Tag Filter Dropdown to the Users Admin Screen
To enhance user management, we can add a filter dropdown to the WordPress Users admin screen, enabling administrators to filter users based on the custom “User Tags” taxonomy.
/**
* Add a dropdown filter for 'User Tags' on the Users admin screen.
*
* @param string $which The location of the extra table nav markup: 'top' or 'bottom'.
*/
function gq_add_user_tag_filter_dropdown( $which ) {
global $pagenow;
// Only add the filter on the main Users admin page, and at the top of the table
if ( 'users.php' !== $pagenow || 'top' !== $which ) {
return;
}
// Retrieve all terms in the 'user_tag' taxonomy
$tags = get_terms( [
'taxonomy' => 'user_tag',
'hide_empty' => false,
] );
// If no tags are found or there's an error, exit the function
if ( empty( $tags ) || is_wp_error( $tags ) ) {
return;
}
// Check if a specific tag is selected in the filter
$selected = isset( $_GET['user_tag_filter'] ) ? (int) $_GET['user_tag_filter'] : 0;
?>
<label for="user_tag_filter" class="screen-reader-text">
<?php esc_html_e( 'Filter by User Tag', 'growquest' ); ?>
</label>
<select name="user_tag_filter" id="user_tag_filter">
<option value="0"><?php esc_html_e( 'Filter by user tags...', 'growquest' ); ?></option>
<?php foreach ( $tags as $tag ) : ?>
<option value="<?php echo esc_attr( $tag->term_id ); ?>"
<?php selected( $selected, $tag->term_id ); ?>>
<?php echo esc_html( $tag->name ); ?>
</option>
<?php endforeach; ?>
</select>
<?php
submit_button( __( 'Filter' ), 'button', null, false );
}
add_action( 'manage_users_extra_tablenav', 'gq_add_user_tag_filter_dropdown' );
/**
* Filter the user query to include users with the selected 'User Tag'.
*
* @param WP_User_Query $query The current WP_User_Query instance.
*/
function gq_filter_users_by_user_tag( $query ) {
global $pagenow;
// Only modify the query on the main Users admin page
if ( ! is_admin() || 'users.php' !== $pagenow ) {
return;
}
// Check if a specific 'user_tag_filter' is set in the request
if ( empty( $_GET['user_tag_filter'] ) ) {
return; // No specific tag selected
}
// Get the selected tag ID
$tag_id = (int) $_GET['user_tag_filter'];
// Retrieve user IDs associated with the selected 'user_tag' term
$user_ids = get_objects_in_term( $tag_id, 'user_tag' );
// If no users are found for this term, set to an array with a non-existent user ID to return no results
if ( empty( $user_ids ) ) {
$user_ids = [ 0 ];
}
// Modify the user query to only include users with the selected 'user_tag'
$query->set( 'include', $user_ids );
}
add_action( 'pre_get_users', 'gq_filter_users_by_user_tag' );
Explanation:
This code introduces a dropdown filter to the Users admin screen, allowing administrators to filter users by the custom “User Tags” taxonomy. The gq_add_user_tag_filter_dropdown
function adds the dropdown at the top of the Users admin page, populated with all available user tags retrieved using get_terms()
. When a specific tag is selected and the filter is applied, the gq_filter_users_by_user_tag
function modifies the user query to display only users associated with the selected tag. This is achieved by hooking into the pre_get_users
action and adjusting the query parameters accordingly. This enhancement streamlines user management by providing administrators with the ability to quickly filter users based on custom taxonomy terms.
Complete Code
Below is the complete solution for categorizing WordPress users with custom taxonomies. Copy and add this code to your child-theme’s functions.php
file or a site-specific plugin. To maintain best practices and avoid conflicts, all functions are prefixed with gq_
.
/**
* Register custom taxonomy 'User Tags' for WordPress users.
*/
function gq_register_user_tag_taxonomy() {
register_taxonomy( 'user_tag', 'user', [
'labels' => [
'name' => _x( 'User Tags', 'Taxonomy General Name', 'growquest' ),
'singular_name' => _x( 'User Tag', 'Taxonomy Singular Name', 'growquest' ),
'menu_name' => __( 'User Tags', 'growquest' ),
'all_items' => __( 'All User Tags', 'growquest' ),
'parent_item' => __( 'Parent User Tag', 'growquest' ),
'parent_item_colon' => __( 'Parent User Tag:', 'growquest' ),
'new_item_name' => __( 'New User Tag', 'growquest' ),
'add_new_item' => __( 'Add New User Tag', 'growquest' ),
'edit_item' => __( 'Edit User Tag', 'growquest' ),
'update_item' => __( 'Update User Tag', 'growquest' ),
'view_item' => __( 'View User Tag', 'growquest' ),
'add_or_remove_items' => __( 'Add or Remove User Tags', 'growquest' ),
'choose_from_most_used' => __( 'Choose from Most Used User Tags', 'growquest' ),
'popular_items' => __( 'Popular User Tags', 'growquest' ),
'search_items' => __( 'Search User Tags', 'growquest' ),
'not_found' => __( 'Not Found', 'growquest' ),
'no_terms' => __( 'No User Tags', 'growquest' ),
'items_list' => __( 'User Tags List', 'growquest' ),
'items_list_navigation' => __( 'User Tags List Navigation', 'growquest' ),
],
'hierarchical' => false, // set to true for categories-like taxonomy, false for tags-like
'public' => false,
'show_ui' => true, // allows management via admin dashboard
'show_admin_column' => false,
'show_in_nav_menus' => false,
'show_tagcloud' => false,
'rewrite' => false,
] );
}
// Hook into WordPress init action.
add_action( 'init', 'gq_register_user_tag_taxonomy' );
/**
* Add 'User Tags' taxonomy management page under the 'Users' menu.
*/
function gq_add_user_tags_admin_page() {
$taxonomy = get_taxonomy( 'user_tag' );
add_users_page( esc_attr( $taxonomy->labels->menu_name ), esc_attr( $taxonomy->labels->menu_name ), $taxonomy->cap->manage_terms, 'edit-tags.php?taxonomy=' . $taxonomy->name );
}
add_action( 'admin_menu', 'gq_add_user_tags_admin_page' );
/**
* Ensure the 'Users' menu is highlighted when editing 'User Tags' taxonomy terms.
*
* @param string $parent_file Current parent file.
*
* @return string Modified parent file.
*/
function gq_fix_user_tags_parent_file( $parent_file ) {
global $submenu_file;
if ( isset( $_GET['taxonomy'] ) && 'user_tag' === $_GET['taxonomy'] && 'edit-tags.php?taxonomy=user_tag' === $submenu_file ) {
$parent_file = 'users.php';
}
return $parent_file;
}
add_filter( 'parent_file', 'gq_fix_user_tags_parent_file' );
/**
* Modify columns for the 'User Tags' taxonomy admin screen.
* Removes the default 'Posts' column and adds a custom 'Users' column.
*
* @param array $columns Existing columns.
*
* @return array Modified columns array.
*/
function gq_manage_user_tag_taxonomy_columns( $columns ) {
// Remove default 'Posts' count column.
unset( $columns['posts'] );
// Add new 'Users' column.
$columns['users'] = __( 'Users', 'growquest' );
return $columns;
}
add_filter( 'manage_edit-user_tag_columns', 'gq_manage_user_tag_taxonomy_columns' );
/**
* Populate custom 'Users' column with user count for each term.
*
* @param string $content Existing column content.
* @param string $column_name The current column name.
* @param int $term_id Term ID.
*
* @return string Modified column content.
*/
function gq_populate_user_tag_taxonomy_column( $content, $column_name, $term_id ) {
if ( 'users' === $column_name ) {
$term = get_term( $term_id, 'user_tag' );
if ( $term && ! is_wp_error( $term ) ) {
$content = esc_html( $term->count );
} else {
$content = '0';
}
}
return $content;
}
add_filter( 'manage_user_tag_custom_column', 'gq_populate_user_tag_taxonomy_column', 10, 3 );
/**
* Adds a 'User Tags' column to the main WordPress Users admin page.
*
* @param array $columns Existing columns in Users admin.
*
* @return array Modified columns with 'User Tags' added.
*/
function gq_add_user_tags_column( $columns ) {
// Insert 'User Tags' after the 'Username' column.
$offset = array_search( 'username', array_keys( $columns ), true ) + 1;
return array_slice( $columns, 0, $offset, true ) + [ 'user_tags' => __( 'User Tags', 'growquest' ) ] + array_slice( $columns, $offset, null, true );
}
add_filter( 'manage_users_columns', 'gq_add_user_tags_column' );
/**
* Display assigned 'User Tags' terms for each user in the custom column.
*
* @param string $output Default column output.
* @param string $column_name Current column name.
* @param int $user_id The user ID.
*
* @return string Modified column content.
*/
function gq_populate_user_tags_column( $output, $column_name, $user_id ) {
if ( 'user_tags' === $column_name ) {
$terms = wp_get_object_terms( $user_id, 'user_tag' );
if ( ! empty( $terms ) && ! is_wp_error( $terms ) ) {
$names = wp_list_pluck( $terms, 'name' );
$output = implode( ', ', $names );
} else {
$output = '—';
}
}
return $output;
}
add_filter( 'manage_users_custom_column', 'gq_populate_user_tags_column', 10, 3 );
/**
* Display 'User Tags' multi-select field on user profile edit screen.
*
* @param WP_User $user The current WP_User object.
*/
function gq_display_user_tags_field( $user ) {
$taxonomy = get_taxonomy( 'user_tag' );
if ( ! current_user_can( $taxonomy->cap->assign_terms ) ) {
return;
}
$user_tags = get_terms( [ 'taxonomy' => 'user_tag', 'hide_empty' => false ] );
?>
<h3><?php esc_html_e( 'User Tags', 'growquest' ); ?></h3>
<table class="form-table">
<tr>
<th><label for="user_tag_select"><?php esc_html_e( 'Select User Tags', 'growquest' ); ?></label></th>
<td>
<?php if ( ! empty( $user_tags ) && ! is_wp_error( $user_tags ) ) : ?>
<select name="user_tag[]" id="user_tag_select" multiple style="min-width:250px;">
<?php
foreach ( $user_tags as $tag ) :
$selected = false;
// If editing an existing user
if ( is_object( $user ) && ! empty( $user->ID ) ) {
$selected = is_object_in_term( $user->ID, 'user_tag', $tag->term_id );
}
?>
<option value="<?php echo esc_attr( $tag->term_id ); ?>" <?php selected( $selected ); ?>>
<?php echo esc_html( $tag->name ); ?>
</option>
<?php endforeach; ?>
</select>
<?php else : ?>
<p><?php esc_html_e( 'No user tags found.', 'growquest' ); ?></p>
<?php endif; ?>
</td>
</tr>
</table>
<?php
}
add_action( 'user_new_form', 'gq_display_user_tags_field' );
add_action( 'show_user_profile', 'gq_display_user_tags_field' );
add_action( 'edit_user_profile', 'gq_display_user_tags_field' );
/**
* Save the selected 'User Tags' when user profile is updated.
*
* @param int $user_id The ID of the current user being edited.
*/
function gq_save_user_tags_field( $user_id ) {
$taxonomy = get_taxonomy( 'user_tag' );
// Permission checks
if ( ! current_user_can( 'edit_user', $user_id ) || ! current_user_can( $taxonomy->cap->assign_terms ) ) {
return;
}
// Assign selected terms
if ( ! empty( $_POST['user_tag'] ) ) {
$terms = array_map( 'absint', $_POST['user_tag'] );
wp_set_object_terms( $user_id, $terms, 'user_tag', false );
} else {
// Remove existing terms if none selected
wp_delete_object_term_relationships( $user_id, 'user_tag' );
}
}
add_action( 'user_register', 'gq_save_user_tags_field' );
add_action( 'personal_options_update', 'gq_save_user_tags_field' );
add_action( 'edit_user_profile_update', 'gq_save_user_tags_field' );
/**
* Add a dropdown filter for 'User Tags' on the Users admin screen.
*
* @param string $which The location of the extra table nav markup: 'top' or 'bottom'.
*/
function gq_add_user_tag_filter_dropdown( $which ) {
global $pagenow;
// Only add the filter on the main Users admin page, and at the top of the table
if ( 'users.php' !== $pagenow || 'top' !== $which ) {
return;
}
// Retrieve all terms in the 'user_tag' taxonomy
$tags = get_terms( [
'taxonomy' => 'user_tag',
'hide_empty' => false,
] );
// If no tags are found or there's an error, exit the function
if ( empty( $tags ) || is_wp_error( $tags ) ) {
return;
}
// Check if a specific tag is selected in the filter
$selected = isset( $_GET['user_tag_filter'] ) ? (int) $_GET['user_tag_filter'] : 0;
?>
<label for="user_tag_filter" class="screen-reader-text">
<?php esc_html_e( 'Filter by User Tag', 'growquest' ); ?>
</label>
<select name="user_tag_filter" id="user_tag_filter">
<option value="0"><?php esc_html_e( 'Filter by user tags...', 'growquest' ); ?></option>
<?php foreach ( $tags as $tag ) : ?>
<option value="<?php echo esc_attr( $tag->term_id ); ?>"
<?php selected( $selected, $tag->term_id ); ?>>
<?php echo esc_html( $tag->name ); ?>
</option>
<?php endforeach; ?>
</select>
<?php
submit_button( __( 'Filter' ), 'button', null, false );
}
add_action( 'manage_users_extra_tablenav', 'gq_add_user_tag_filter_dropdown' );
/**
* Filter the user query to include users with the selected 'User Tag'.
*
* @param WP_User_Query $query The current WP_User_Query instance.
*/
function gq_filter_users_by_user_tag( $query ) {
global $pagenow;
// Only modify the query on the main Users admin page
if ( ! is_admin() || 'users.php' !== $pagenow ) {
return;
}
// Check if a specific 'user_tag_filter' is set in the request
if ( empty( $_GET['user_tag_filter'] ) ) {
return; // No specific tag selected
}
// Get the selected tag ID
$tag_id = (int) $_GET['user_tag_filter'];
// Retrieve user IDs associated with the selected 'user_tag' term
$user_ids = get_objects_in_term( $tag_id, 'user_tag' );
// If no users are found for this term, set to an array with a non-existent user ID to return no results
if ( empty( $user_ids ) ) {
$user_ids = [ 0 ];
}
// Modify the user query to only include users with the selected 'user_tag'
$query->set( 'include', $user_ids );
}
add_action( 'pre_get_users', 'gq_filter_users_by_user_tag' );